Table of Contents
VBA For CATIA V5
Like most applications there is a set of API Application Programming Interfaces that allow VB, Visual Basic talk to the application, and navigate its class structure, to the Methods and properties for each class (object). We can use these API’s to build up a macro to process a common or repetitive task.
Visual Basic
If you have never done any programming before then we need to start of at step 1. Let’s start of by imagining a box, the application and inside this box are other boxes each other box may contain additional boxes inside etc. Not only does each box contain other boxes but also has attributes or properties and methods, actions that box can perform.
The boxes we will refer to as objects (Classes) which have methods and properties. A property could be the Name of the object or the Color, or the count of other boxes contained within. A method maybe Close, Open, Print or Add New Object.
Object Types
So now we understand the basic hierarchy of the object class lets discuss types of Objects. There are three types of objects Abstract, Collections and Objects.
Abstract objects, are base classes (Objects) that other derived classes inherit from. For example, a Base Object would be a Document Object, derived from this would be Part Document, Drawing Document and Product Document. This is important, because we may want to write a macro that works across multiple document types, we don’t want to call out a specific type, because then the script will only work with this type. so we need to use the abstract object since it can be any document type. this is due to all of the document types inheriting this base class and then extending, to create a new derived type.
Collection objects, are collections of a specific type or abstract type. Essentially they are lists, that we can iterate through for example the Documents object is a collection of Document. Notice the Plural verses the Singular this will be important later on.
Finally, we have simple objects, all object types will have Properties and Methods.
Object Navigation
If we go back to the start of the Post we spoke about the Application being a Box of boxes, next we need to know how to traverse from one box to another so we can access the correct Methods and Property’s associated to that box.
To navigate from one box to another we simple place a period between the current box to the one contained within for example Catia.Documents we traversed from the Catia Application object to the Documents Object then we can use the methods and Property’s within the Documents object, this is also done by using a period between the Object and the Method or Property you want to use. For Example to get the number of open documents we can use the Count property Catia.Documents.Count this will return an Integer of the number of open documents.
Managing Memory
The application when running is in memory but the data is stored in a format that can only be accessed by that application and in addition we don’t know where in memory that data is stored. So with a language like VBA Visual Basic for Applications we have to create our own space in memory and write our own data to that empty space in memory.
This is done with three keywords Dim, As and Set, and will be done in two steps creating the space in memory and then setting the space in memory, sounds difficult but lets look at a simple case.
Dim ioCATIA As Application
Here we can see the first two key word Dim and As, so what are the other two words in this statement, ioCATIA is the name for the space in memory also referred to as the variable name. This can more or less be anything as long as it doesn’t start with a number or special character and contain spaces and, or special charters. The last word Application is the TypeName of the object, every object in the object hierarchy has a TypeName and we need to know what these are so we can easily navigate this structure. This hierarchy of TypeName’s can be found in the automation documents for the application.
Once we have the space in memory created, we need to populate it with our own reference to the object using the Set keyword.
Set ioCATIA = CATIA
The very top object is the CATIA object which has a TypeName of Application, atypically the object name and TypeName are identical. The Set keyword allows us to fill a space in memory of a given type with an object of that type by using the ‘=‘ equals symbol.
When we start doing some examples this will make sense.
Main Routine
With all macros, there has to be something called a routine this is a collection code lines that can be executed sequentially. To tell the computer that this block of code is a routine we have to declare a header and a footer.
Sub CATMain() End Sub
The key word Sub indicates to the computer that this is a sub-routine the next word is the name of the Sub-Routine, with CATIA the primary Sub-Routine or the entry point must be called CATMain. At the end of line are two parentheses (), these allow us to pass data into the Sub-Routine but well cover this much later on.
Finally the End Sub, which tells the computer that the end of the code has been reached.
Function Vs. Sub-Routine
So we have seen a simple Sub-Routine the CATMain, there is a second type of Routine called a Function. The difference between a Sub-Routine and a Function is that a Sub-Routine does a task, whereas a Function does a Task and Returns some data of a given type.
Before we saw that a Sub-Routine is structured as below;
Sub CATMain() End Sub
A Function is very similar;
Function NewPart() As Document End Function
Both Sub-Routine and Function can accept arguments between the Parenthesis, however a Type is declared after the Parenthesis when a Function is created. This is very similar to the Dim statement where the As TypeName is also used. What is happening is the Function name becomes a Variable of the given Type in this case Document.
So we have to Equate a Variable of the same Type to the Function, when we want to call the Function from a Sub-Routine.
Sub CATMain() Dim ioDocument as Document Set ioDocument = GetDocument() End Sub Function GetDocument() as Document End Function
We can expand on this and pass in a variable to the Function, in this case we can pass a string into the function as long as we declare that we will be passing in a string to the function. This is also very similar to Declaring a Variable, we have the Variable Name and Variable Type within the Functions Parenthesis.
Sub CATMain() Dim ioDocument as Document Set ioDocument = GetDocument("Part") End Sub Function GetDocument(iType As String) as Document End Function
Simple Program
So we know we must have the Sub CATMain() with the End Sub as the starting point, we also know that we have to get the application object.
Sub CATMain() Dim ioCATIA As Application Set ioCATIA = CATIA End Sub
Now we have the CATIA object, we can access its Methods and Property’s or navigate to one of the related objects, but how do we know what Methods, Properties or Objects are? In the installation path;
C:\Program Files\Dassault Systemes\B20\win_b64\code\bin
You will find a .chm file named V5Automation.chm. When this file is opened, the following page can be seen.
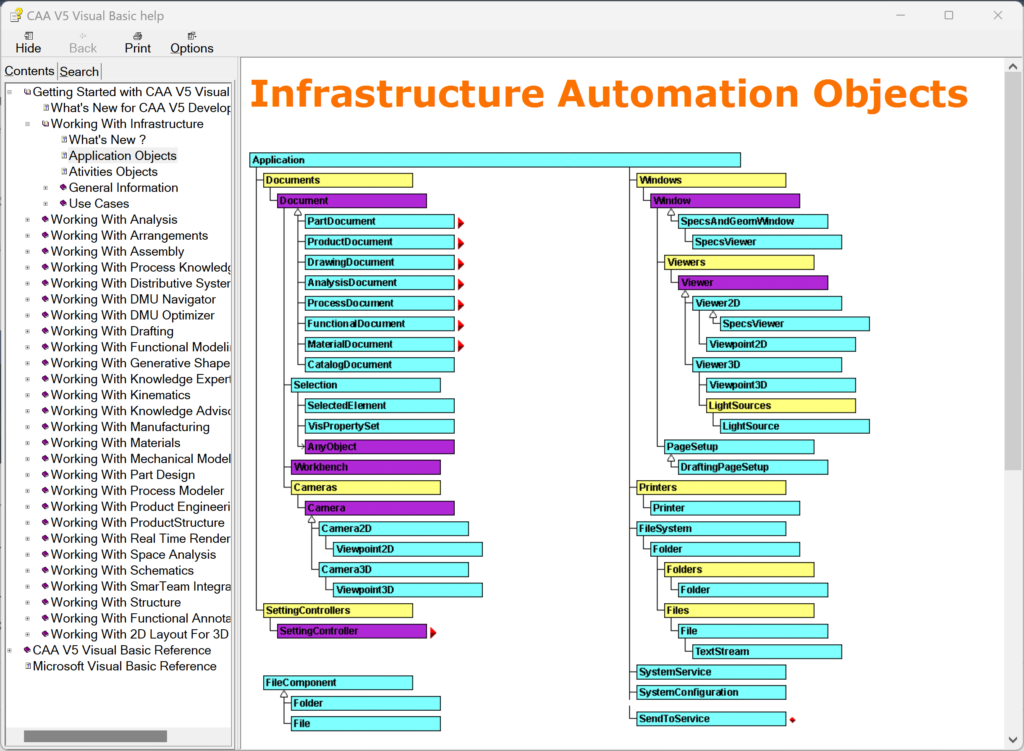
The hierarchy displayed on the right shows the object TypeNames, and based on their color the, if the object is an Abstract (Purple), Collection (Yellow) or Regular object (Cyan).
We can now navigate down the hierarchy and access each objects Methods and Properties. So, lets Navigate down to the Documents Collection and Create a New Part, Product and Drawing.
Sub CATMain() Dim ioCATIA As Application Set ioCATIA = CATIA Dim ioDocuments as Documents Set ioDocuments = ioCATIA.Documents End Sub
Each Dim Statement allows us to create an Empty space in memory with a give Variable Name and Type.
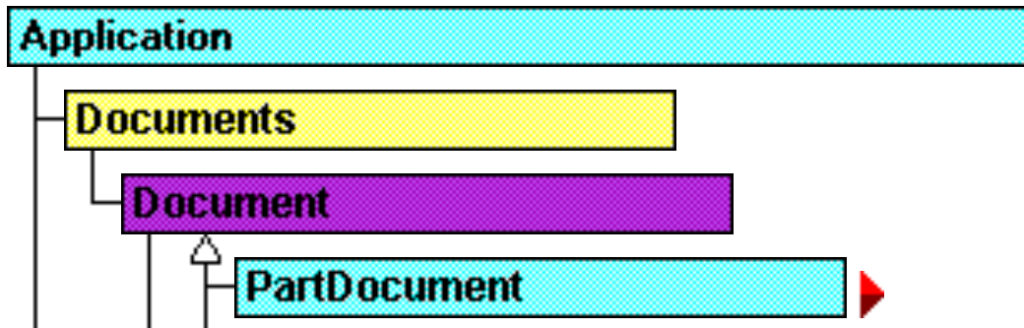
We can clearly see that the two object types we created were; Application and Documents. When we select on the Object in the .Chm we can quickly navigate to the Methods and Properties the Object has.
The Method Index for Document contains Add, Item, NewFrom, Open and Read, as shown below.
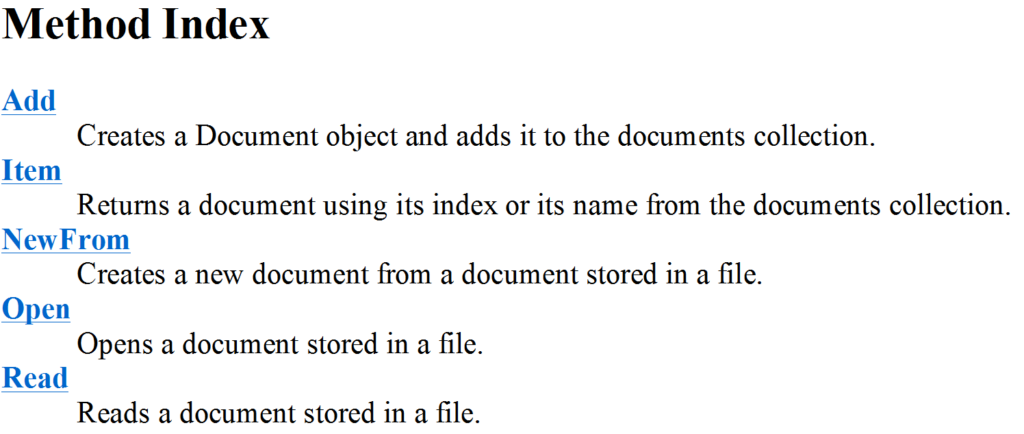
When one of these is selected, typically an example will be shown. Below we can see the Add Method. So lets dissect this a little bit. We can see that the method is a Function, so we know that it returns something, and by looking at the TypeName after the As keyword we know its a Document type. Finally, within the perenthisis we can see we need to pass in a variable of Type CATBSTR aka String for the docType. Within the documentation it tells you what three docTypes we are allowed to use; Part, Product and Drawing,a nd it aslo shows a simple example.
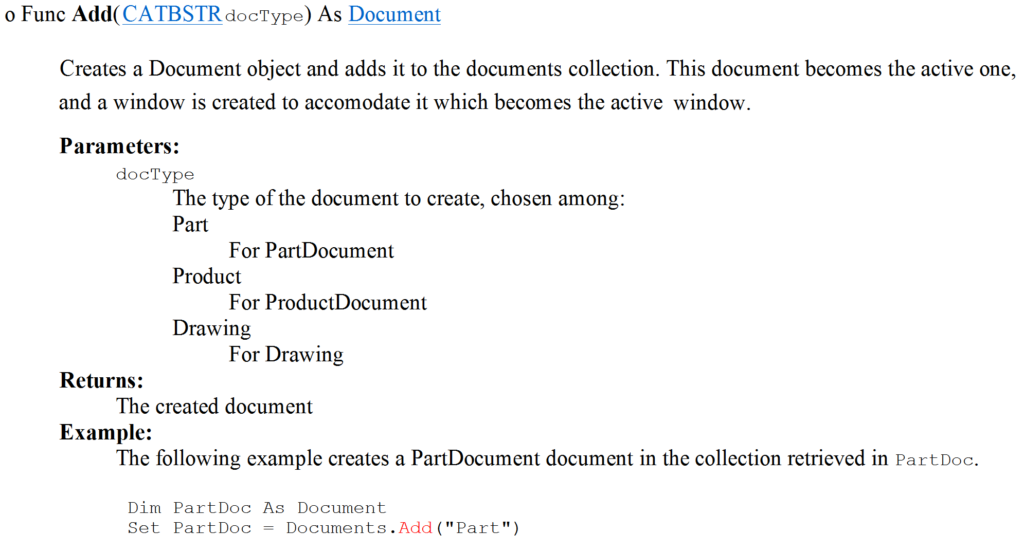
So we can now finish our script.
Sub CATMain() Dim ioCATIA As Application Set ioCATIA = CATIA Dim ioDocuments as Documents Set ioDocuments = ioCATIA.Documents Dim ioPartDocument as Document Set ioPartDocument = ioDocuments.Add("Part") Dim ioProductDocument As Document Set ioProductDocument = ioDocuments.Add("Product") Dim ioDrawingDocument as Document Set ioDrawingDocument = ioDocuments.Add("Drawing") End Sub
This will absolutely work, however each time we used the abstract object Document. This means when we start using the VBA Editor we wont see all of the Methods, and Properties that belong to the specific Types PartDocument, ProductDocument and DrawingDocument. This is because the specific Types inherit from the Document Type and then are Extended with specific Methods and Properties for that Extended Type.
So lets fix that.
Sub CATMain() Dim ioCATIA As Application Set ioCATIA = CATIA Dim ioDocuments as Documents Set ioDocuments = ioCATIA.Documents Dim ioPartDocument as PartDocument Set ioPartDocument = ioDocuments.Add("Part") Dim ioProductDocument As ProductDocument Set ioProductDocument = ioDocuments.Add("Product") Dim ioDrawingDocument as DrawingDocument Set ioDrawingDocument = ioDocuments.Add("Drawing") End Sub