Securing App Settings With Azure Functions
In the last post we connected the function to a MySql database, by reading the elements of a connection string from the Azure Key Vault. However were still hardcoding the Key Vault URI and the Names of the Secrets in the code behind. We need to remove these and Azure has away in which to manage this data and its done in two ways. While were developing we add the information into the local.settings,json file, but this file does not get deployed to Azure, so we must also create these variables and their values in Azure within the Application Settings vault in Azure and that’s what were going to do in the post.
public static class PeopleFunctions { public static readonly string kvUri = $"https://catiawidget-blah.blah.azure.net/"; public static readonly List<string> secrets = new List<String> { "DBConnectionURI", "DBDatabase", "DBUserName", "DBUserPassword" }; [FunctionName("GetAllPeople")]
Local Setting Json
When we created the Function in Visual Studio a local.settings,json file was created with a couple of variables.
{ "IsEncrypted": false, "Values": { "AzureWebJobsStorage": "UseDevelopmentStorage=true", "FUNCTIONS_WORKER_RUNTIME": "dotnet" } }
Were going to add two more variables to this file and then change the two read only static variables to read from the local.settings,json, the KeyVault_URI and the Secret_Names and their associated values.
{ "IsEncrypted": false, "Values": { "AzureWebJobsStorage": "UseDevelopmentStorage=true", "FUNCTIONS_WORKER_RUNTIME": "dotnet", "KeyVault_URI": "https://catiawidget-blah.blah.azure.net/", "Secret_Names": "DBConnectionURI,DBDatabase,DBUserName,DBUserPassword" } }
In the Function class we can tweak our two static property’s to read from this file.
namespace DemoFunctionApp { public static class PeopleFunctions { //These must be added in Azure in the AppSettings Section and local.settings.json public static readonly string kvUri = Environment.GetEnvironmentVariable("KeyVault_URI"); public static readonly List<string> secrets = Environment.GetEnvironmentVariable("Secret_Names").Split(',').ToList(); [FunctionName("GetAllPeople")]
However as previously mentioned the local.settings.json is not published, so we also have to create the same variables in Azure.
Azure Function Variables
Within Azure navigate to the function and select the Configuration menu item, then select New Application Setting.
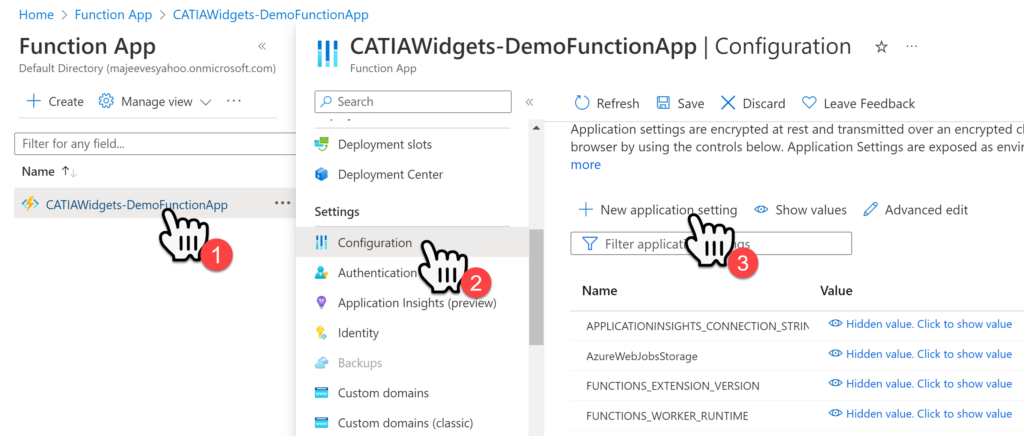
Within the Add Edit Application Setting page key in a Name and a Value then select Ok. Repeat this for the setting ensuring that the Name and Values are identical to the ones in the local.settings,json file.
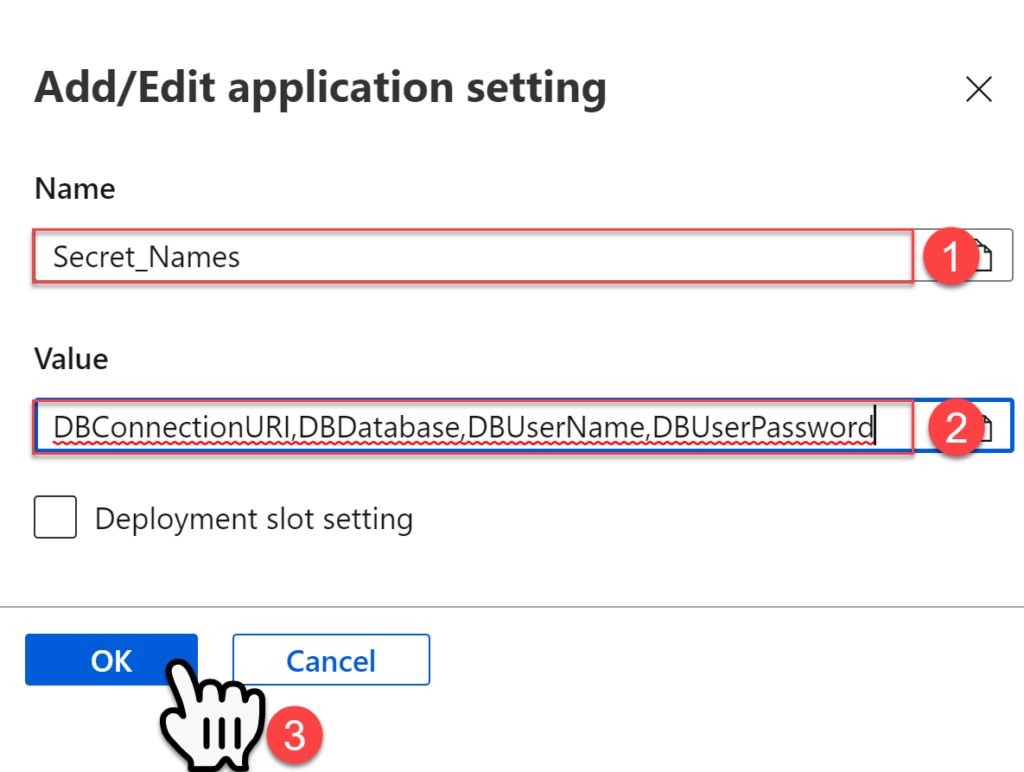
Once both application settings have been created, select Save in the Configuration panel.
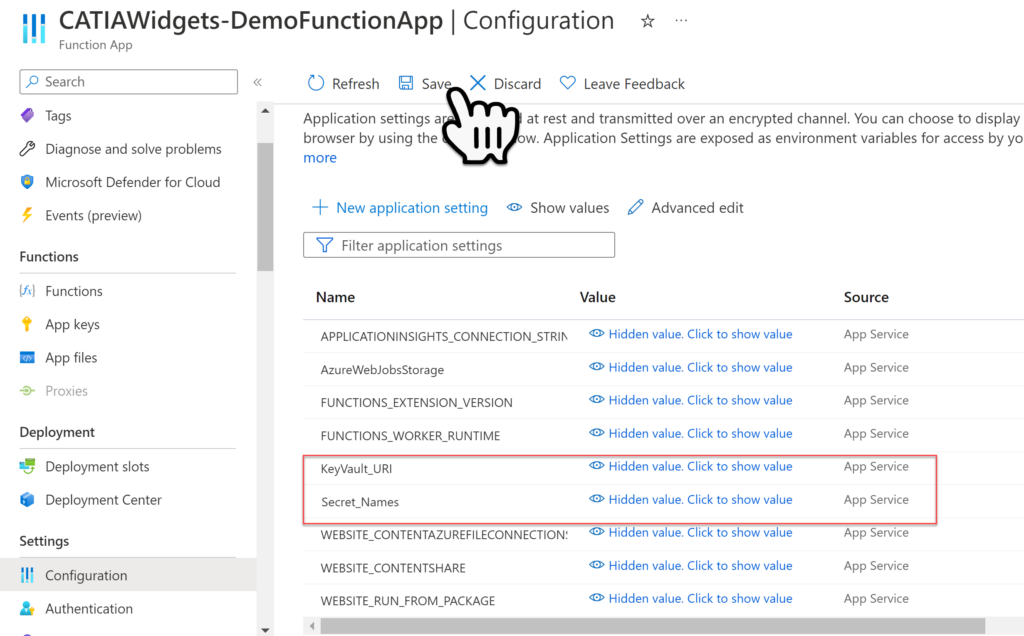
When the Save Changes warning message is shown select Continue.
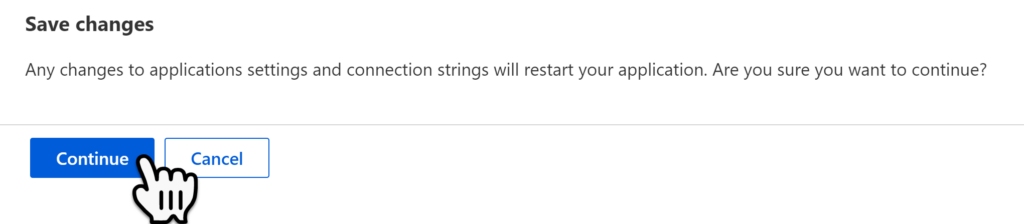
When we deploy and run the function the application settings will now be secure.