Table of Contents
C# Code Documentation
Just a quick post
In the world of software development, writing code is just one part of the equation. Equally important is ensuring that your code is well-documented. Code documentation serves as a guide for developers, making it easier to understand and maintain the codebase. In this post, we’ll explore the importance of code documentation and best practices for writing effective documentation.
Why Code Documentation Matters
- Understanding Complex Logic: Code can be intricate, especially in large projects. Documentation helps developers grasp the logic behind the code without having to decipher every line.
- Onboarding New Developers: When new team members join a project, thorough documentation can significantly reduce their ramp-up time. Clear explanations and examples make it easier for them to understand the codebase and start contributing effectively.
- Facilitating Collaboration: In collaborative environments, documentation promotes better communication among team members. It ensures that everyone is on the same page regarding code functionality and usage.
- Maintenance and Debugging: Well-documented code is easier to maintain and debug. When issues arise, developers can quickly locate the relevant sections of code and understand their purpose, speeding up the debugging process.
Best Practices for Code Documentation
- Use Descriptive Names: Meaningful variable names, function names, and comments can convey the intent of your code. Avoid cryptic abbreviations and acronyms.
- Write Clear Comments: Comment not just what your code does, but why it does it. Explain the rationale behind decisions and any potential gotchas. Use inline comments sparingly, focusing on areas of complexity or ambiguity.
- Embrace Documentation Tools: Utilize tools like XML documentation in C#, Javadoc in Java, or Doxygen for multiple languages. These tools generate documentation from specially formatted comments, ensuring consistency and ease of maintenance.
- Provide Examples: Include code examples demonstrating how to use your functions and classes. Real-world examples help developers understand usage patterns and edge cases.
- Update Documentation Regularly: Code evolves over time, and so should its documentation. Whenever you make changes to your code, ensure that the corresponding documentation is updated accordingly.
- Document Interfaces and Public APIs: For libraries and frameworks, comprehensive documentation of public interfaces is crucial. Clearly specify inputs, outputs, and behavior to guide users effectively.
- Consider the Audience: Tailor your documentation to the intended audience. Internal APIs may require less detailed documentation than public-facing APIs or open-source projects.
Examples of Code Documentation Tags in C#
Summary Tag:
/// <summary> /// Adds two numbers together. /// </summary> /// <param name="a">The first number.</param> /// <param name="b">The second number.</param> /// <returns>The sum of the two numbers.</returns> public int Add(int a, int b) { return a + b; }
Remark Tag:
/// <remarks> /// This method assumes that both numbers are positive integers. /// </remarks> public int AddPositiveNumbers(int a, int b) { if (a < 0 || b < 0) { throw new ArgumentException("Both numbers must be positive."); } return a + b; }
Example Tag:
/// <example> /// This example shows how to use the Add method. /// <code> /// int result = Add(2, 3); /// Console.WriteLine($"Result: {result}"); // Output: Result: 5 /// </code> /// </example>
Code Tag:
/// <summary> /// Adds two numbers together. /// </summary> /// <param name="a">The first number.</param> /// <param name="b">The second number.</param> /// <returns>The sum of the two numbers.</returns> public int Add(int a, int b) { return a + b; } /// <remarks> /// This method assumes that both numbers are positive integers. /// </remarks> public int AddPositiveNumbers(int a, int b) { if (a < 0 || b < 0) { throw new ArgumentException("Both numbers must be positive."); } return a + b; } /// <example> /// This example shows how to use the Add method. /// <code> /// int result = Add(2, 3); /// Console.WriteLine($"Result: {result}"); // Output: Result: 5 /// </code> /// </example>
Conclusion
Code documentation is not just an afterthought; it’s a fundamental aspect of software development. Investing time in writing clear, concise, and comprehensive documentation pays off in the long run by enhancing code readability, maintainability, and collaboration. By following best practices and making documentation a priority, you can ensure that your code is not just functional but also understandable to others.
Remember, good code documentation isn’t just about making your code readable; it’s about empowering developers to build upon and maintain your work effectively. So, the next time you write code, don’t forget to document it—it’s an investment in the future of your project and your team’s success.
When using the methods in Visual studio, we will now see a well structured popup due to the mark up language previously discussed.
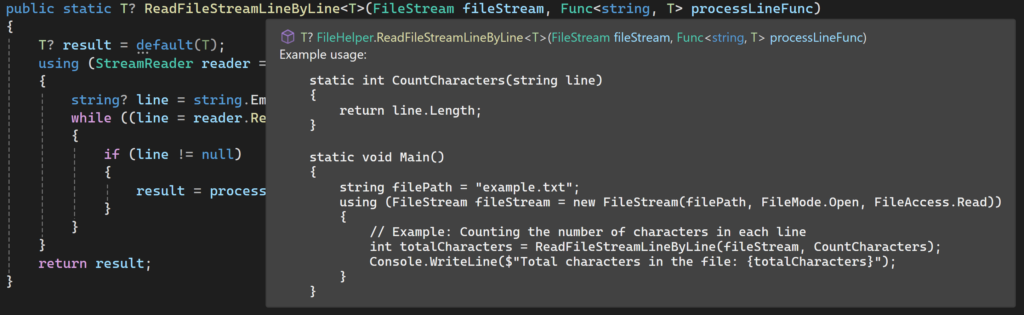