Table of Contents
C# Create a WPF Splash Screen
In this post we will create a simple Splash Screen for a WPF application.
Assets
Within the Visual Studio project create a folder called Assets with a sub folder called Images/ Once this is done add a .png file called BackGround.png, ensure you set the properties Build Action to Resources and Copy to Output Directory to Copy if Newer.
Materials Design
First we want to add a Nuget Package called Materials Design, this can be easily done by editing the Project.cs file and adding the following Package Reference.
<ItemGroup> <PackageReference Include="MaterialDesignThemes" Version="4.9.0" /> </ItemGroup>
Once this is done we can update the App.xaml file by adding the following Application Resources.
<Application.Resources> <ResourceDictionary> <ResourceDictionary.MergedDictionaries> <ResourceDictionary Source="pack://application:,,,/MaterialDesignThemes.Wpf;component/Themes/MaterialDesignTheme.Light.xaml" /> <ResourceDictionary Source="pack://application:,,,/MaterialDesignThemes.Wpf;component/Themes/MaterialDesignTheme.Defaults.xaml" /> <ResourceDictionary Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Primary/MaterialDesignColor.DeepPurple.xaml" /> <ResourceDictionary Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Accent/MaterialDesignColor.Lime.xaml" /> </ResourceDictionary.MergedDictionaries> </ResourceDictionary> </Application.Resources>
Splash Screen Window
Next we will add a new XAML Window called SplashScreen to the project.
SplashScreen.xaml
Next we will edit the SplashScreen.xaml file, to layout the splash screen.
<Window x:Class="WPFSplashScreen.SplashScreen" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:WPFSplashScreen" xmlns:materialDesign="http://materialdesigninxaml.net/winfx/xaml/themes" mc:Ignorable="d" Title="SplashScreen" Height="470" Width="750" WindowStyle="None" WindowStartupLocation="CenterScreen" AllowsTransparency="True" Background="{x:Null}" ContentRendered="Window_ContentRendered"> <materialDesign:Card UniformCornerRadius="15" Background="{DynamicResource MaterialDesignPaper}" materialDesign:ElevationAssist.Elevation="Dp4" Margin="25"> <Grid> <Grid.ColumnDefinitions> <ColumnDefinition Width="350"/> <ColumnDefinition Width="400"/> </Grid.ColumnDefinitions> <StackPanel Grid.Column="0" HorizontalAlignment="Left" VerticalAlignment="Center" Margin="50,0,0,0"> <WrapPanel> <Image Source="Assets/Images/BackGround.png" HorizontalAlignment="Left" Height="50" Width="50"></Image> <TextBlock Text="TrueNorth PLM" FontSize="28" FontWeight="Bold" HorizontalAlignment="Left" VerticalAlignment="Center" Margin="15,0,0,0"> </TextBlock> </WrapPanel> <TextBlock Text="Loading Reources" FontSize="17" FontWeight="SemiBold" HorizontalAlignment="Left" Margin="0,30,0,15"/> <ProgressBar Name="progressBar" Value="0" Height="5" Width="280" IsIndeterminate="True"/> </StackPanel> <StackPanel Grid.Column="1" HorizontalAlignment="Left" VerticalAlignment="Center" Height="320" Width="320"> <Image Source="Assets/Images/BackGround.png" Width="320" Height="320"/> </StackPanel> </Grid> </materialDesign:Card> </Window>
SplashScreen.xaml.cs
The code below manages the length of time the splash screen is displayed, after which it will close the splash screen and then display the main window.
using System; using System.ComponentModel; using System.Threading; using System.Windows; namespace WPFSplashScreen { public partial class SplashScreen : Window { public SplashScreen() { InitializeComponent(); } private void Window_ContentRendered(object sender, EventArgs e) { BackgroundWorker worker = new BackgroundWorker(); worker.WorkerReportsProgress = true; worker.DoWork += worker_DoWork; worker.ProgressChanged += worker_ProgressChanged; worker.RunWorkerAsync(); } private void worker_DoWork(object? sender, DoWorkEventArgs e) { for (int i = 0; i <= 100; i++) { if (sender is BackgroundWorker) { BackgroundWorker worker = (BackgroundWorker)sender; worker.ReportProgress(i); Thread.Sleep(80); } } } private void worker_ProgressChanged(object? sender, ProgressChangedEventArgs e) { progressBar.Value = e.ProgressPercentage; if(progressBar.Value ==100) { MainWindow mainWindow = new MainWindow(); Close(); mainWindow.ShowDialog(); } } } }
StartUp URI
Finally we can change the StartUpUri to point to the new Splash Screen window.
<Application x:Class="WPFSplashScreen.App" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="clr-namespace:WPFSplashScreen" StartupUri="SplashScreen.xaml">
Executing the Code
When the code executes we should first see the following Splash Screen then the Main Window shortly afterwards.
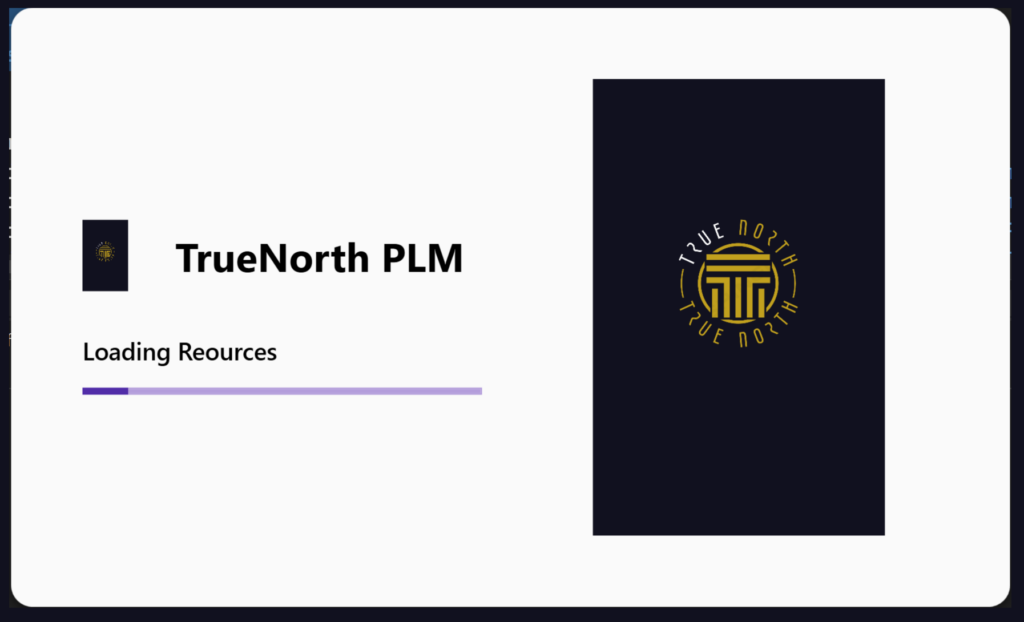