Determines the Correct Boolean Orientations for a Corner Operation
With an EKL corner operation the 5th and 6th Arguments are the Normal orientations of the Supporting Curves. There are four possible combinations that could be correct;
- True : True
- True : False
- False : True
- False : False
And in addition these are inverted based on the supports orientation as well, so we have three Normal’s to take into account, in order to derive the correct Boolean values;
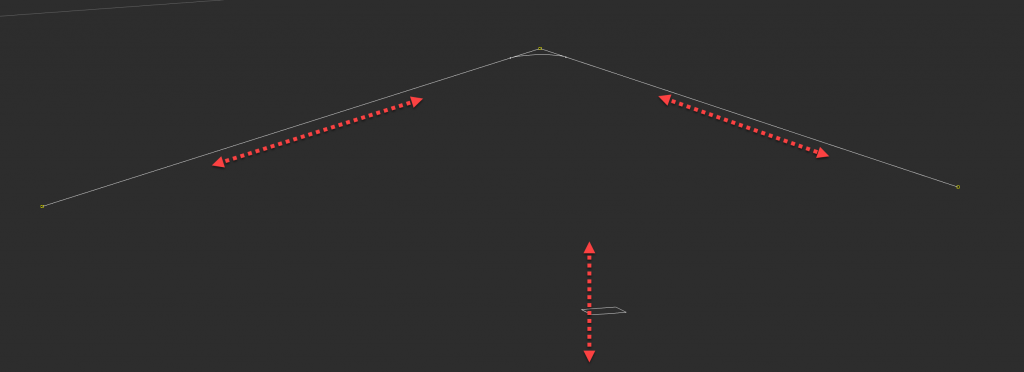
This makes writing a rule tricky, below is an action that determines the correct orientations for the two curves being trimmed into a corner.
// Determines the Correct Boolean Orientations for a corner operation // corner( iCurve1 , iCurve2 , `xy plane` , 5mm , ioBoolean1 , ioBoolean2 , True ) // iCurve1 : Curve // iCurve2 : Curve // iPlane : Plane // oBoolean1 : Boolean // oBoolean2 : Boolean // DeterminCornerOrientations( iCurve1 : Curve , iCurve2 : Curve , iPlane : Plane ) oBoolean1 : Boolean , oBoolean2 : Boolean Let iIntersection , iSetBack1 , iSetBack2 , iSetBackMidPoint( Point ) Let iCircle ( Circle ) Let iSetBackLine , iTangLine1 , iTangLine2 , iTestLine ( Line ) Let iCorner1 , iCorner2 , iCorner3 , iCorner4 ( Curve ) iIntersection = intersect( iCurve1 , iCurve2 ) iCircle = circleCtrRadius( iIntersection , iPlane , 1mm , 1 , 0deg , 180deg ) iSetBack1 = intersect( iCurve1 , iCircle ) iSetBack2 = intersect( iCurve2 , iCircle ) iSetBackLine = line( iSetBack1 , iSetBack2 ) iSetBackMidPoint = pointoncurveRatio( iSetBackLine , NULL , 0.5 , True ) iTestLine = line( iIntersection , direction( line( iIntersection , iSetBackMidPoint )) , 0mm , 1000mm , True ) iTangLine1 =linetangent( iCurve1 , iIntersection , 10mm , -10mm , True ) iTangLine2 =linetangent( iCurve2 , iIntersection , 10mm , -10mm , True ) // The corners have to be differant sizes for the near to work iCorner1 = corner( iTangLine1 , iTangLine2 , iPlane , 1mm , True , True , False ) iCorner2 = corner( iTangLine1 , iTangLine2 , iPlane , 0.9mm , True , False , False ) iCorner3 = corner( iTangLine1 , iTangLine2 , iPlane , 0.8mm , False , True , False ) iCorner4 = corner( iTangLine1 , iTangLine2 , iPlane , 0.7mm , False , False , False ) // Use this to get the correct untrimmed corner curve // Let AssembledCorner ( Curve ) // AssembledCorner = assemble( iCorner1 , iCorner2 , iCorner3 , iCorner4 ) // Let oCurve ( Curve ) // oCurve = near( AssembledCorner , iTestLine ) // // Using the end points of the corner the support curves can be trimmed and assembled. // Let ioCornerStart, ioCornerEnd ( Point ) // ioCornerStart = pointoncurveRatio( oCurve , NULL , 0 , True ) // ioCornerEnd = pointoncurveRatio( oCurve , NULL , 1 , True ) // // Let ioSplit1, ioSplit2 ( Curve ) // ioSplit1 = split( iCurve1, ioCornerStart , True ) // ioSplit2 = split( iCurve2, ioCornerEnd , True ) // // ioSplit1 = ( distance( iIntersection , ioSplit1 ) <> 0mm )? ioSplit1 ; split( iCurve1, ioCornerStart , False ) // ioSplit2 = ( distance( iIntersection , ioSplit2 ) <> 0mm )? ioSplit2 ; split( iCurve2, ioCornerEnd , False ) // oCurve = assemble( ioSplit1 , oCurve , ioSplit2 ) If ( distance( iCorner1 , iTestLine ) == 0mm ) { oBoolean1 = True oBoolean2 = True } Else If ( distance( iCorner2 , iTestLine ) == 0mm ) { oBoolean1 = True oBoolean2 = False } Else If ( distance( iCorner3 , iTestLine ) == 0mm ) { oBoolean1 = False oBoolean2 = True } Else { oBoolean1 = False oBoolean2 = False }
If we want to directly create the corner we can do a simpler way however we will be changing the Normal’s of the input curves and creating the support plane dynamically.
// Creates a Corner by Changing the inpu Curve Normals to a Standard and Creating the Support Plane // iCurve1 : Curve // iCurve2 : Curve // iRadius : Length // oCurve : Curve // CreateCorner( iCurve1 : Curve , iCurve2 : Curve , iRadius : Length ) oCurve : Curve Let iIntersection , iEndPoint ( Point ) Let iPlane ( Plane ) Set iIntersection = intersect( iCurve1 , iCurve2 ) iEndPoint = pointoncurveRatio( iCurve1 , NULL , 0 , True ) If ( distance( iIntersection , iEndPoint ) == 0mm ) { Set iCurve1 = invert( iCurve1 ) } iEndPoint = pointoncurveRatio( iCurve2 , NULL , 0 , True ) If ( distance( iIntersection , iEndPoint ) == 0mm ) { Set iCurve2 = invert( iCurve2 ) } iPlane = plane( linetangent( iCurve1 , iIntersection , 0mm , 10mm , True ) , linetangent( iCurve2 , iIntersection , 0mm , 10mm , True ) ) oCurve = corner( iCurve1 , iCurve2 , iPlane , iRadius , False , True , True )