Table of Contents
#5 Logging Within My Lambda Function
Before we get to much further and our code gets to complicated, we probably should look at adding some sort of logging within AWS. There are ways in which you can run your code locally for debugging purposes and we will look into this approach later on, but for now we want to leverage AWS Cloud Watch and create a log group specifically for our Lambda function.
appsettings.json
Within the appsettings.json file we want to add some additional JSON which is in two main sections; Logging, and Serilog. There are many logging packages that you can use with AWS and are supported by AWS but Serilog is probably the best one. The Logging section just defines that fact that we want to log some information. Since we’re using Serilog, we have to add a Serilog section that defines it’s AWS usage. This section also allows us to define a custom Log Group which will essentially categorize our logs into a single group and make them easy to find.
So lets add the definition as shown below, you can change the ‘LogGroup’ and ‘CustomLogGroup’ names as required.
{ "Logging": { "LogLevel": { "Default": "Information" } }, "Serilog": { "Region": "eu-west-1", "LogGroup": "MyFirstLambda_AppLog", "CustomLogGroup": "MyFirstLambda_AppLog", "LogLevel": 4, "RetentionPolicy": 5 }, "AllowedHosts": "*", "AppS3Bucket": "", "AWSProfileName": "AWS Default" }
NuGet Packages
To make this work we need to add two additional NuGet packages; Serilog.AspNetCore, and Serilog.Sinks.Console.
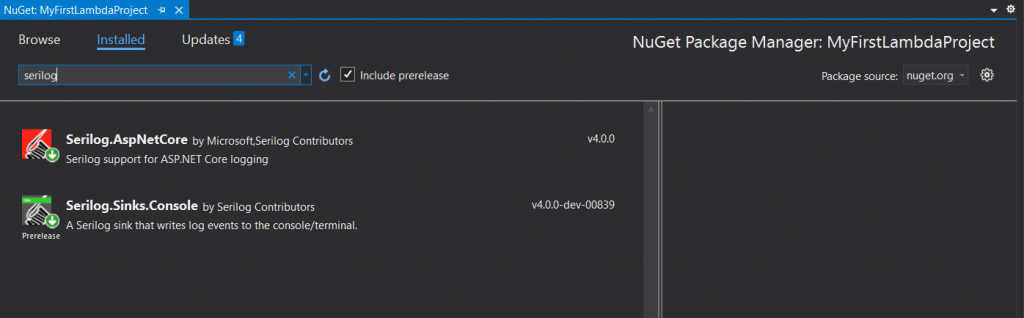
This is achieved by right- mouse clicking on the project and selecting Manage NuGet Packages… from the contextual menu.
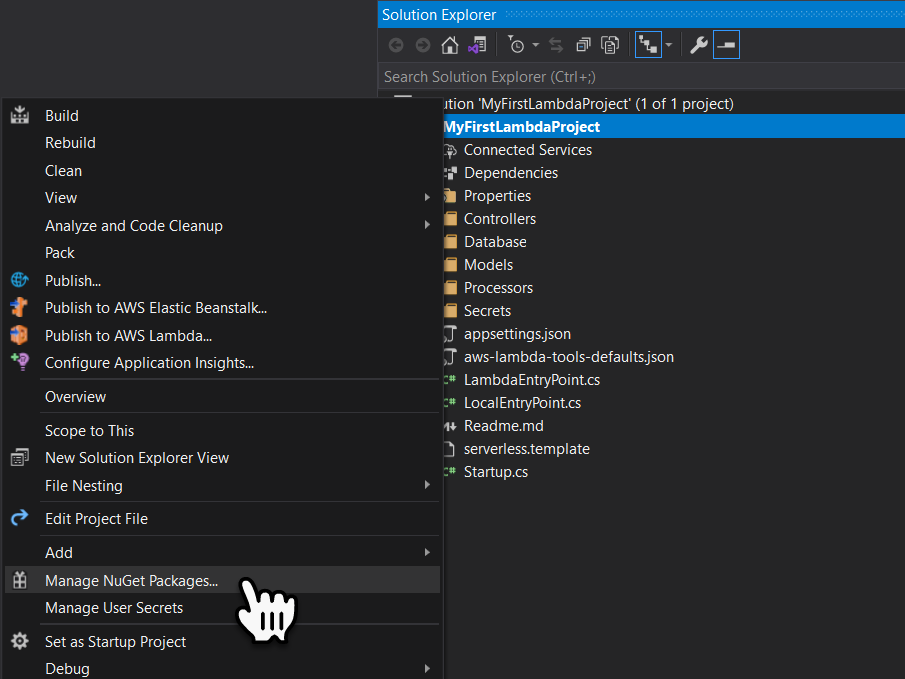
Within the Browse tab key-in ‘Serilog’ and press enter. A list of all the Serilog Packages will be shown.
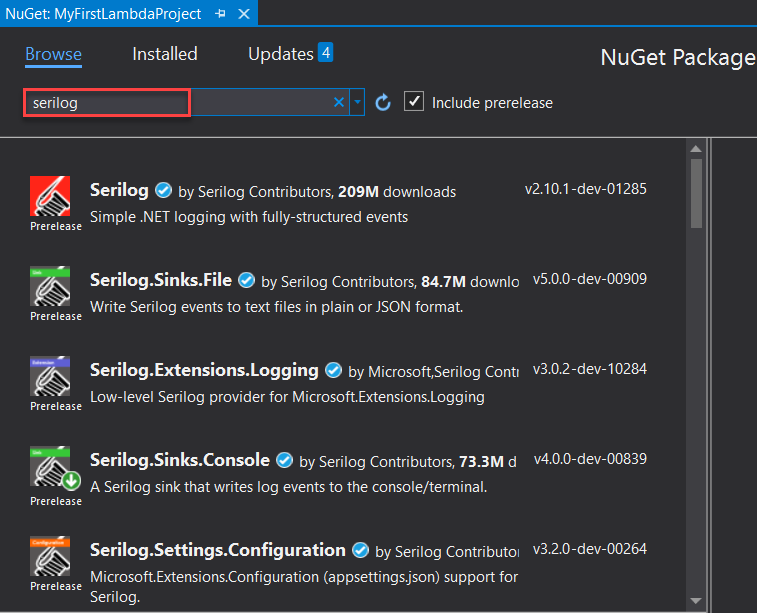
From this list select a package and then select ‘Install’.
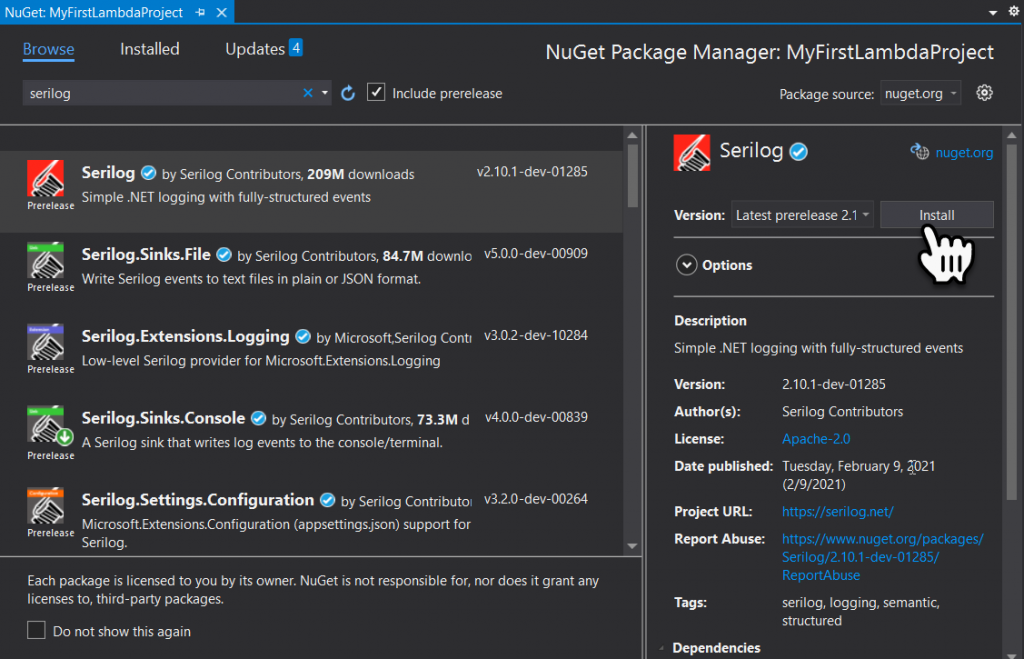
Then follow the prompts. Repeat this process for all of the required Serilog Packages.
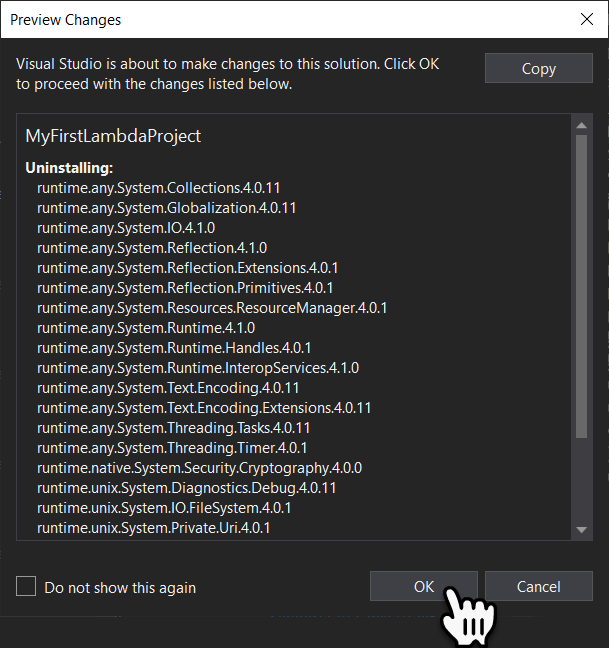
Startup.cs
Within the Startup.cs file add the ‘Using’ reference for Serilog and then within the Startup constructor add the Log.Logger section of code as shown below.
using Serilog; public Startup(IConfiguration configuration) { Configuration = configuration; Log.Logger = new LoggerConfiguration() .Enrich.FromLogContext() .MinimumLevel.Information() .WriteTo.Console() .CreateLogger(); }
Processor
Within the Processor class which contains the methods for each controller end point. We will add a ‘Using’ reference to Serilog again and then create an instance of ILogger. We can then start logging Information, Warnings, or Errors, in the example below the instance of ILogger has also been passed to the ‘DataRepo’ constructor.
using Serilog; public class DataModelProcessor : IDataModelProcessor { private readonly DataRepo _DataRepo = null; private ILogger _log = null; public DataModelProcessor() { _log = Log.Logger; _log.Information("Creating New Data Model Processor."); _DataRepo = new DataRepo(_log); } }
Once we have implemented the logging through the code we must again publish the project to Lambda and using Postman, call one of the API endpoints. Then we can go to AWS Cloud Watch to review the log in the newly created log group.
AWS Cloud Watch
Now we have provided a way in which we can record log-able events to AWS Cloud Watch and made a request to one of our end points we should now be able to review the log in the custom log group. To do this, let’s log back into the AWS Services Console, and Navigate to Cloud Watch.
Within the Cloud Watch console select the Log Groups link in the sidebar menu.
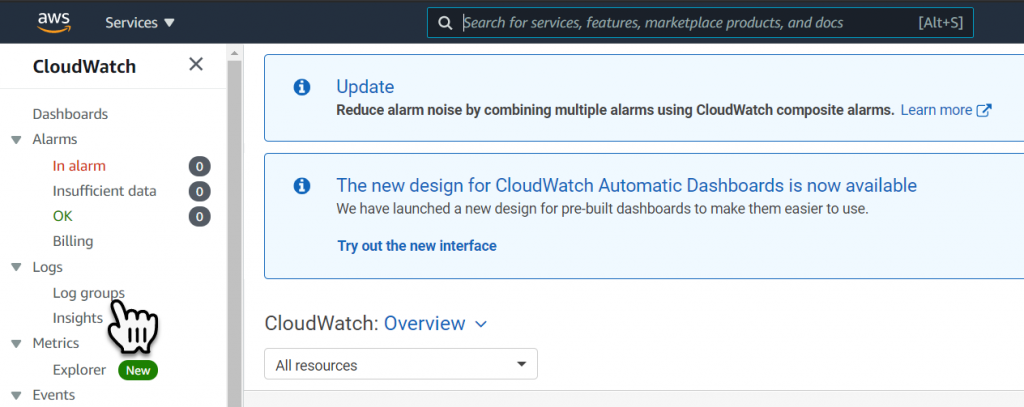
Within the Log Groups we will then select the Log Group that we created.
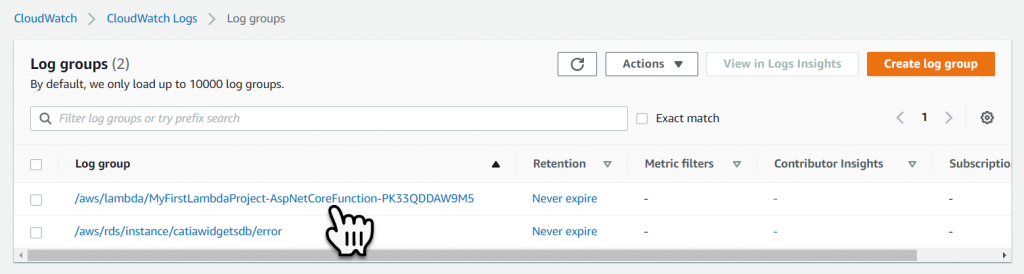
The Log Group will contain one or more Log Streams, select the Latest Log Stream.
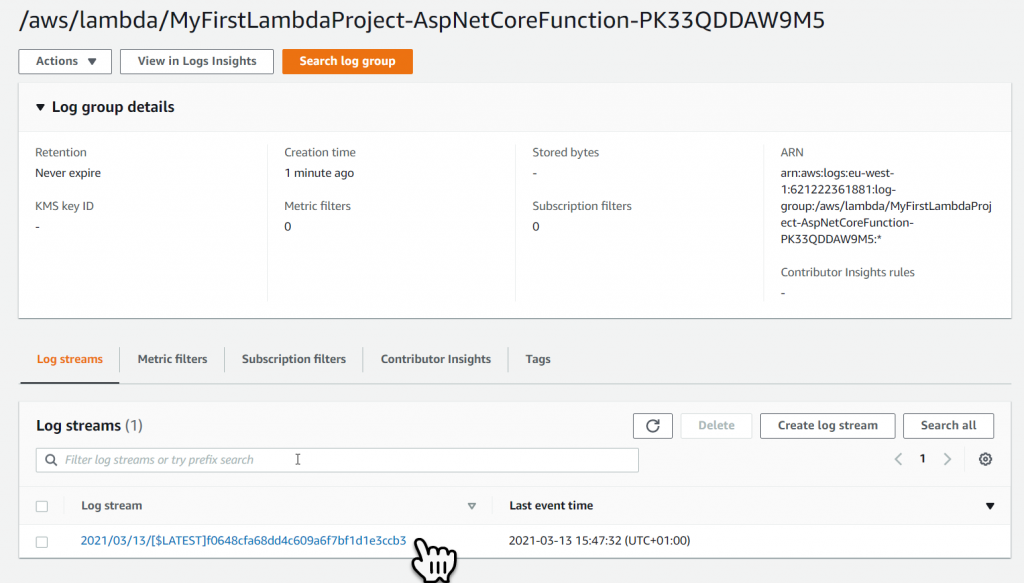
Within the selected Log Stream, we can see some of the Logged Information. Now we can log Information, Errors, or Warnings we can easily debug our code once we start to create the database connection and start making MySQL calls.
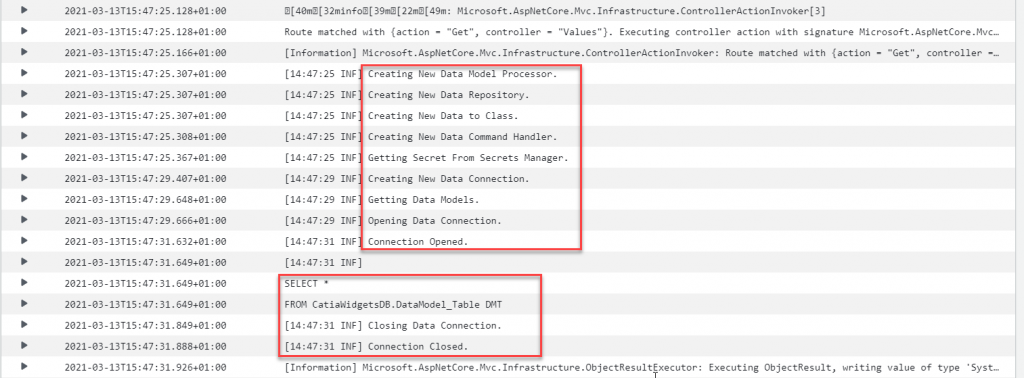